Q6. How to specify the initial value of the Hashmap in JDK 1.8?
Simply use the following declaration.
new HashMap<>(n);
Q7. How to ensure the initial value is 2 to the power of n?
In order to ensure the initial value is 2 to the power of n, we have to leverage an algorithm that first finds the bit with a value of 1 and then changes the following bits to 1.
// Returns a power of two size for the given target capacity.
static final int tableSizeFor(int cap) {
int n = cap - 1;
n |= n >>> 1;
n |= n >>> 2;
n |= n >>> 4;
n |= n >>> 8;
n |= n >>> 16;
return (n < 0) ? 1 : (n >= MAXIMUM_CAPACITY) ? MAXIMUM_CAPACITY : n + 1;
}
Q8: When does HashMap increase its size?
Given a load factor of 0.75, if the default capacity is 16, then when the element reaches 16 x 0.75=12, the HashMap will increase its size.
static final float DEFAULT_LOAD_FACTOR = 0.75f;
Q9: Why the default load factor is 0.75
As per the comment in the source code of HashMap:
* Ideally, under random hashCodes, the frequency of
* nodes in bins follows a Poisson distribution
* (http://en.wikipedia.org/wiki/Poisson_distribution) with a
* parameter of about 0.5 on average for the default resizing
* threshold of 0.75, although with a large variance because of
* resizing granularity. Ignoring variance, the expected
* occurrences of list size k are (exp(-0.5) * pow(0.5, k) /
* factorial(k)). The first values are:
* 0: 0.60653066
* 1: 0.30326533
* 2: 0.07581633
* 3: 0.01263606
* 4: 0.00157952
* 5: 0.00015795
* 6: 0.00001316
* 7: 0.00000094
* 8: 0.00000006
* more: less than 1 in ten million
In an ideal situation, a random hash code is used. When the expansion threshold (load factor) is 0.75, the frequency of node appearance in the Hash bucket (table) follows a Poisson distribution with an average parameter of 0.5. Ignore the variance, that is, X = λt, P(λt = k), where λt = 0.5, according to the formula:
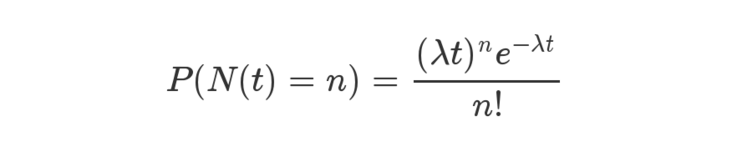
Choosing 0.75 as the default load factor is completely a compromise choice in terms of time and space costs.
Q10: Under what circumstances will the linked list be converted into a red-black tree in JDK 1.8?
static final int TREEIFY_THRESHOLD = 8;